Flocking System
Concept
The concept of Flocking System by Craig Reynolds has been used to do procedural painting with different flocking behaviors. Each agent participating in the flock is an autonomous agent which
1. Has limited ability of perceive their environment
2. Processes the environment and calculates the action
3. Does not have a global plan or leader
​
Just like any living form, the agents will understand their environment based on which they will assess what action to take. Their working style involves three steps, selecting what to do like fleeing, seeking, and path following, turning towards their target position and finally moving towards the target with desired velocity. In short:
​
1. Action Selection
2. Steering
3. Locomotion
While in groups the position, velocity and direction of every agent is influenced by others in its neighborhood at any point in time. Each agent will operate with an awareness of every other agent. The behavior rules that allow agents to stay as a group are:
1. Separation
2. Alignment
3. Cohesion
​
Each one has been implemented individually as well as together as a flock by assigning specific weight to each behavior. Additionally a Path Following algorithm has been applied making the boids trace a specific contour or path.
Painting Patterns with Flocking Rules
Separation ensures that each boid/brush has its own space even though they move together and in close quarters.
Alignment


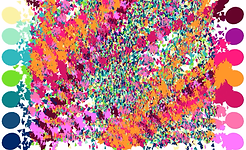
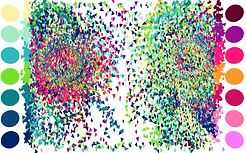
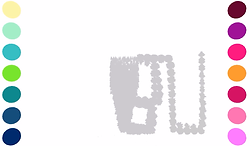
Separation
While painting, when we require multiple colors to flow simultaneously, we hold the brushes with different color together and move them concurrently. Similarly, the boids need to move in same direction and with almost same velocity to produce the individually layered and artistically balanced strokes.
Cohesion
Alignment will move the brushes in one direction, separation will give individual brush its own space, but what compels them to move in unison is cohesion. It makes sure that the brushes trace the path together.
Flocking
All the three behaviors applied simultaneously differing in weights i.e. how much effect each of the behaviors will have on the boids, will give an effect similar to flocking of birds thus carrying a range of colors.
Path Following
Path is a good way when we want the boids to create a specific contour. I used it to make the initials of Bournemouth University, "BU" by setting up target points at the corner locations and making the boids traverse them in sequence.
Implementation
Boids have been made to act as brushes. Initially the flock will start of with no color and will remain in the same mode till they reach a color target location place vertically at either sides from where they will inherit the target's color and move around with the same color till next color target is hit. As soon as the new target is hit, the boids inherit the new target's color which they carry on till next target and so on.
Flocking Behaviours: Separation, Alignment and Cohesion has been implemented using algorithm modified in regard to Daniel Shiffman, Nature of Code (2012).
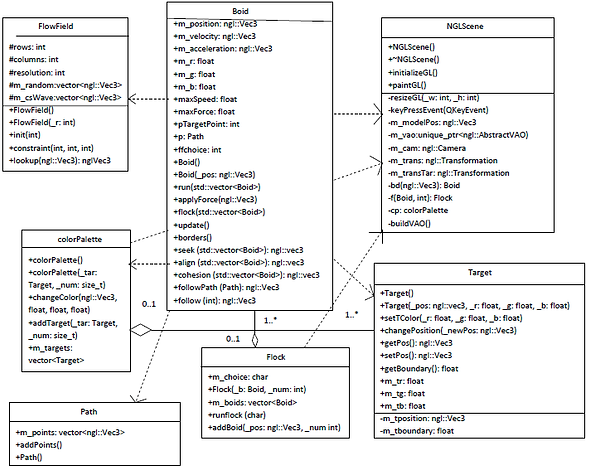
Algorithms
Algorithm: Picking color from the targets
1. for(i from 0 to targetLocations)
2. D = distance between (targetPosition, boid_position)
3. If(D>0 and D<targetBoundary)
4. boid_red = target_red;
5. boid_green = target_green;
6. boid_blue = target_blue
7. endif
8. end
Algorithm: Picking color from the targets
1. for(i from 0 to targetLocations)
2. D = distance between (targetPosition, boid_position)
3. If(D>0 and D<targetBoundary)
4. boid_red = target_red;
5. boid_green = target_green;
6. boid_blue = target_blue
7. endif
8. end
Algorithms
Algorithm: Picking color from the targets
1. for(i from 0 to targetLocations)
2. D = distance between (targetPosition, boid_position)
3. If(D>0 and D<targetBoundary)
4. boid_red = target_red;
5. boid_green = target_green;
6. boid_blue = target_blue
7. endif
8. end
Algorithm: Alignment
1. define boid's neighbor distance
2. find distance between this boid and others
3. if(dist > 0 && dist < neighbor distance)
4. add neighbors' velocities
5. endif
6. desired velocity is average of all neighbor velocities
7. steering = desired - currentVelocity
8. end
Algorithm: Separation
1. set desired separation distance
2. find this boid's and others' distance
3. if(dist > 0 && dist < separation distance)
4. difference = vector pointing away from others' location
5. add difference vector of all neighbors
6. endif
7. desired velocity is average difference
8. steering = desired - currentVelocity
9. end
Algorithm: Cohesion
1. define boid's neighbor distance
2. find this boid's and others' distance
3. if(dist > 0 and dist < neighbor distance)
4. add all neighbor's position
5. endif
6. take average of neighbors' position
7. desired velocity is vector between boid and avgPosition
8. steering = desire - currentVelocity
9. end
Output
Boids have been made to act as brushes. Initially the flock will start of with no color and will remain in the same mode till they reach a color target location place vertically at either sides from where they will inherit the target's color and move around with the same color till next color target is hit. As soon as the new target is hit, the boids inherit the new target's color which they carry on till next target and so on. I wanted to build a GUI to control the number of boids in the flock. to activate a behavior, clear screen and control the number of boids on screen but since I was a bit short of time, I decided to give keyboard control to the user using specific keys.
​
‘s’ or ‘S’ = separation
‘a’ or ‘A’ = alignment
‘c’ or ‘C’ = cohesion
‘f’ or ‘F’ = flocking
‘p’ or ‘P’ = path following
'q' = clear screen